Solana Dev101- How to get the holders of an NFT collection (Snapshot)
Introduction
If you're curious about how to capture snapshots of NFT collection owners, you've come to the right place. With Helius, the process of taking these snapshots is made easy through the use of the DAS API.
To follow along with this tutorial, you'll need a Helius account and API key which you can obtain for free by signing up here. If you have any questions you can refer to the related documentation at the end of the post. Additionally, don't hesitate to join the Helius Discord community or tag Helius on Twitter to ask for further assistance.
Using the DAS API
The Digital Asset Standard (DAS) API is an open-source specification and system that provides a unified interface for interacting with digital assets (tokens, NFTs, etc). We will be using the DAS API to get the owners of specific assets. There are a few different calls that we can use depending on a collection’s method of grouping.
Get NFTs from a collection with a collection address (getAssetByGroup)
The latest version of the Metaplex NFT standard utilizes certified collections. This is achieved by creating an NFT to represent a collection, which allows NFTs to be categorized and for collection information to be verified on the blockchain. To retrieve all the NFTs in a collection, the collection address, which is the mint address of the collection NFT, can be used. If you have an NFT that belongs to a collection, you can look at the NFT's metadata and locate the collection address in the collection section.
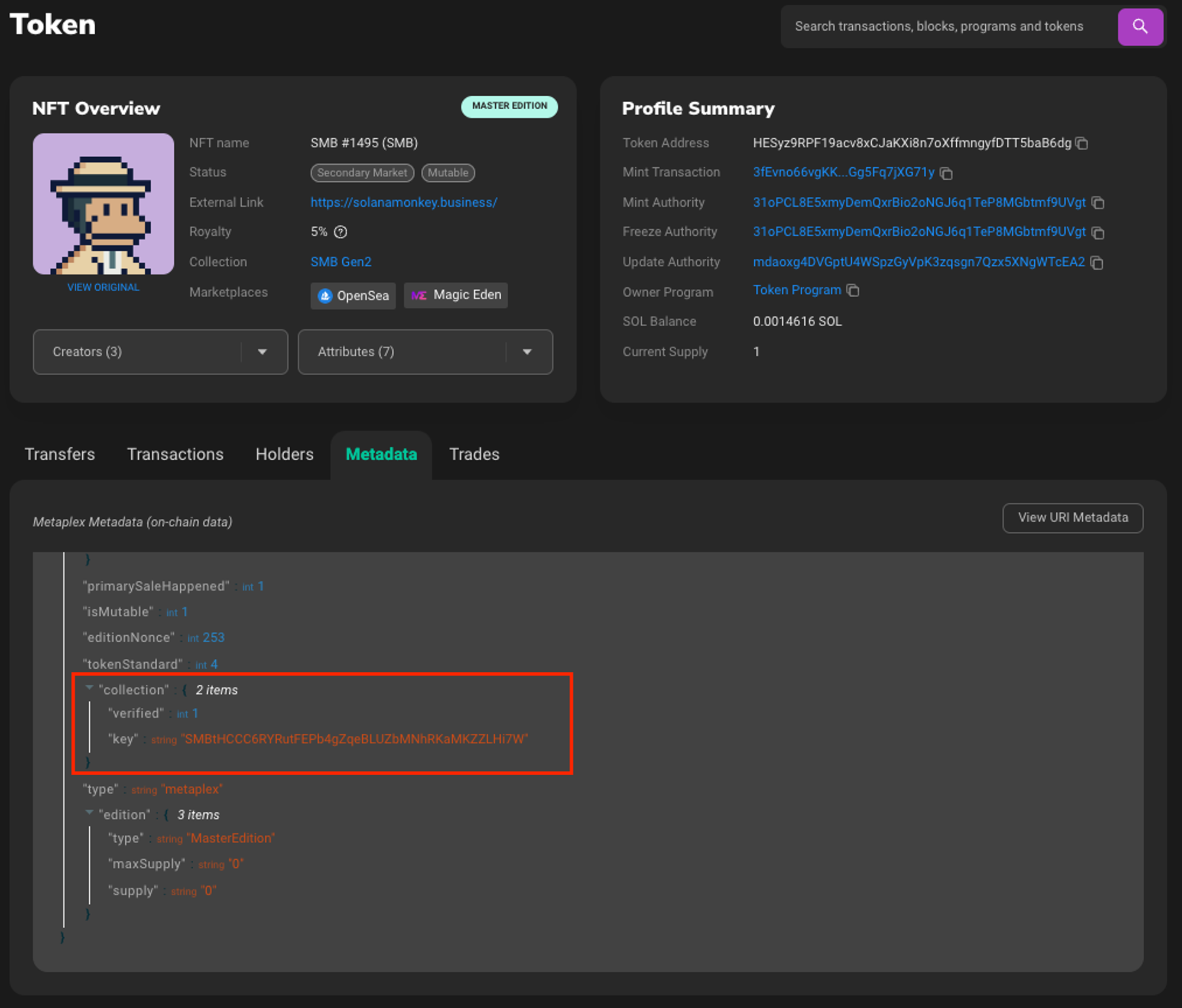
Once you obtain the address, we can use the DAS `getAssetByGroup` method to search for all the NFTs related to it. The following code snippet demonstrates how we can use pagination to iterate through all 10000 Mad Lads NFTs. In the API call's params section, we will specify that we are using a collection as the group key, and we will then provide the collection address as the group value.
Get NFTs from a collection based on the first verified creator (getAssetByCreator)
To take a snapshot with the first verified creator, you need to use the 'getAssetByCreator' function and provide the creator's address in the parameters. You can locate an NFT's creator address in the creators section of its on-chain metadata.
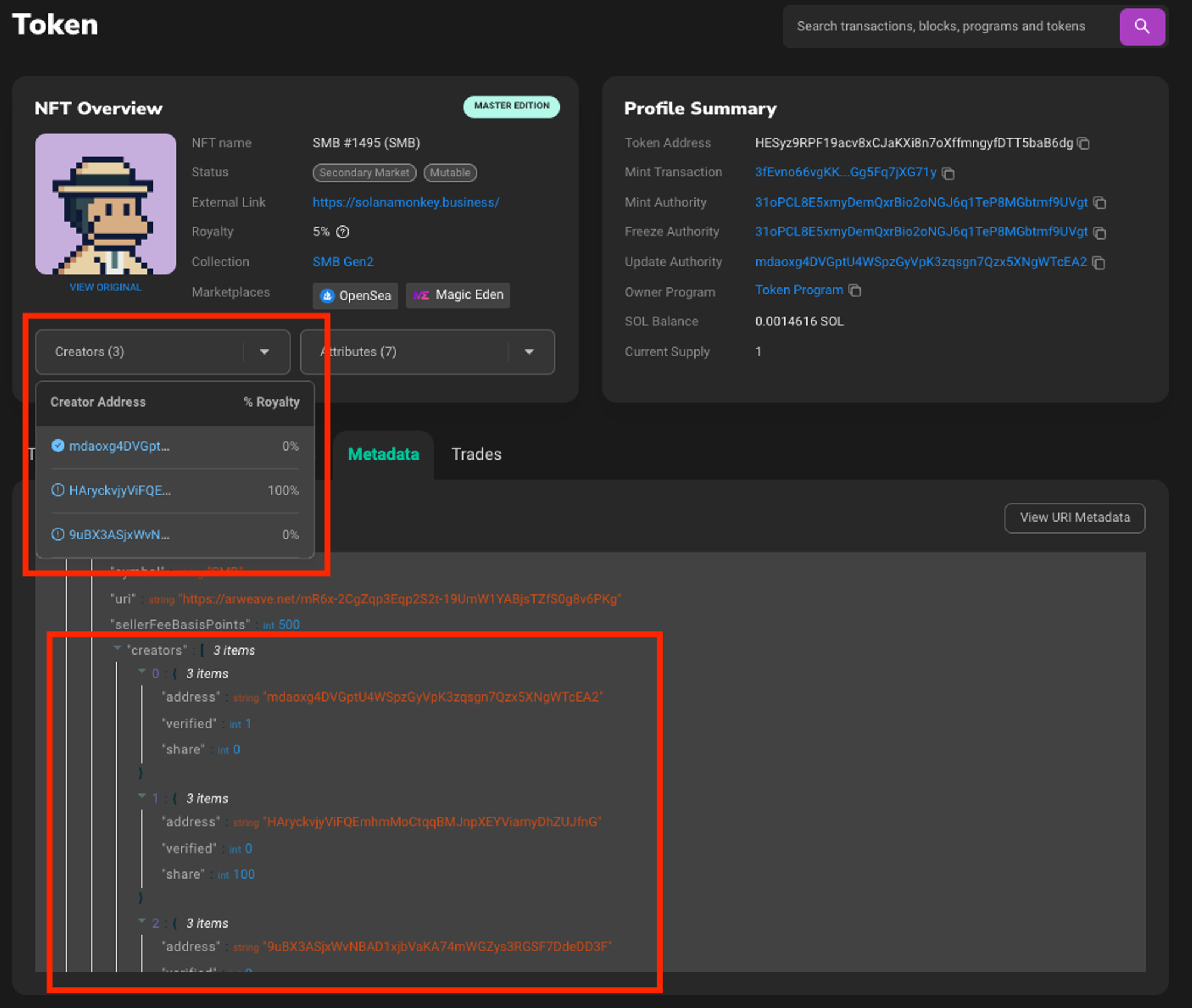
After verifying the first creator, set the address as the creator address in the call to `getAssetsByCreator`.
Output
In all of the code snippets above, we output the address and corresponding owner for each NFT.
Feel free to modify this output to add any necessary information such as the name, description, or attributes. Detailed information about the data that is included in the DAS response can be found in the DAS documentation.
Conclusion
In this blog post, we have discussed how to retrieve information about the holders of an NFT collection using the DAS API. With the help of the DAS API, you can easily obtain valuable information about NFTs and their owners. Whether you want to analyze user behavior or track the distribution of your NFTs, the DAS API provides a convenient solution. You can start using the DAS API today for free by visiting dev.helius.xyz and signing up for a plan.
References
DAS Documentation (Helius): https://docs.helius.dev/compression-and-das-api/digital-asset-standard-das-api