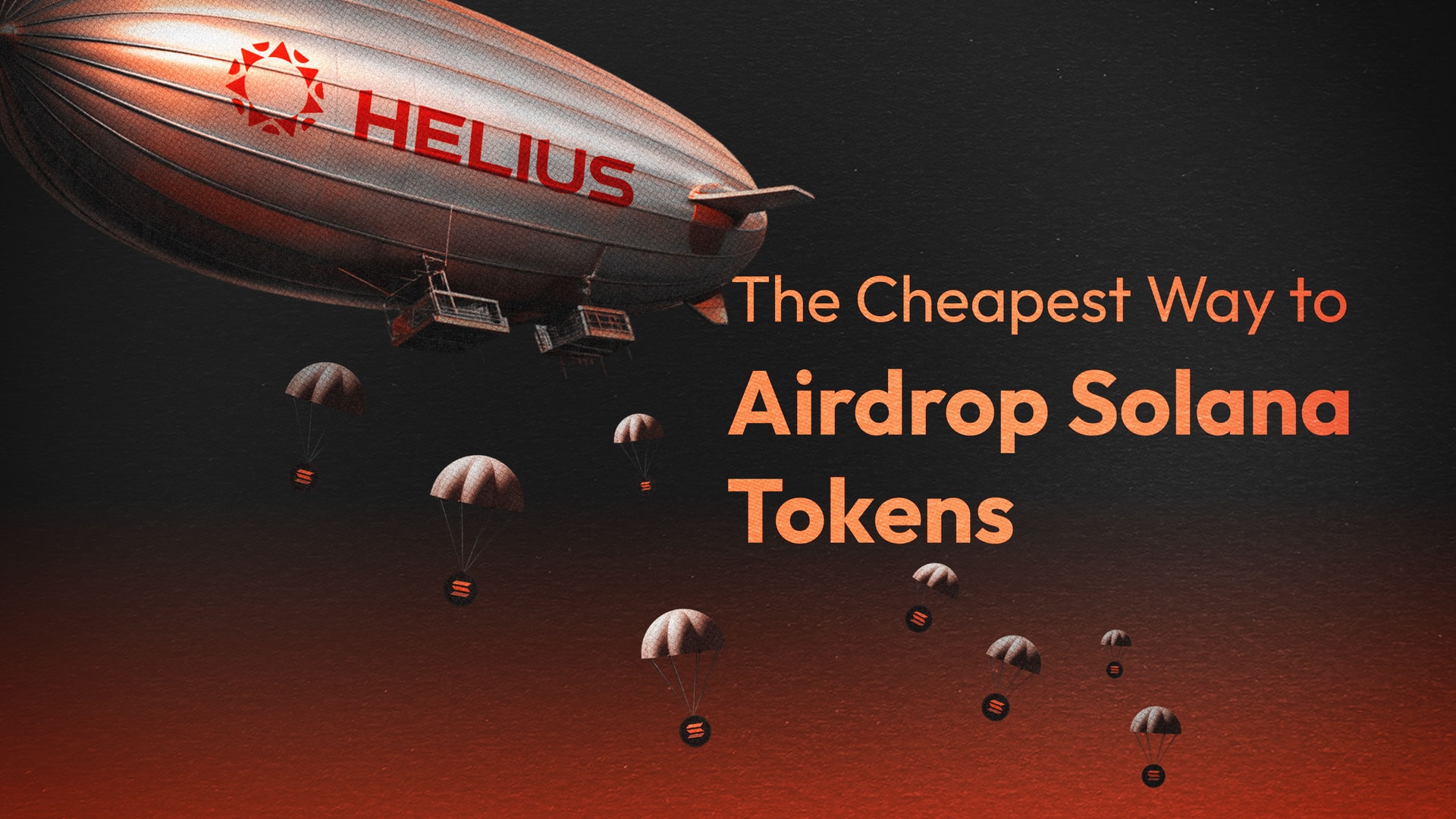
The Cheapest Way to Airdrop Solana Tokens
Airdrops let you send tokens to many people at once. They reward early supporters, attract new users, and help build a strong community. But every transaction has fees it needs to pay, meaning airdrops can become costly rather quickly. On Solana, you may also need to create a token account for each new holder, which drives costs even higher. Once you move beyond a few hundred addresses, these fees can turn what started as an exciting giveaway into a budget headache.
In this guide, we will look at:
- How to do a traditional Solana airdrop
- Where the costs come from
- How zero-knowledge (ZK) compression fixes these problems
- How to use AirShip for cheap, large-scale airdrops
Let's get started.
Traditional Airdrop: How It Works
A standard Solana airdrop requires two steps for each recipient:
- Create an Associated Token Account (ATA), if the recipient doesn’t have one.
- Transfer tokens to that ATA.
Here’s a simplified code example using @solana/web3.js and @solana/spl-token:
// Simple SPL token airdrop script that creates token accounts if they don't exist
import {
Connection,
PublicKey,
Keypair,
Transaction,
sendAndConfirmTransaction,
} from "@solana/web3.js";
import {
createTransferInstruction,
getOrCreateAssociatedTokenAccount,
} from "@solana/spl-token";
import bs58 from 'bs58';
// Configure these values
const TOKEN_MINT = new PublicKey("<YOUR_TOKEN_MINT_ADDRESS>");
const recipients = [
{ address: "<RECIPIENT_WALLET_ADDRESS>", amount: 0.05 }, // amount in tokens (not lamports)
// Add more recipients as needed
];
async function airdropTokens(connection: Connection, senderKeypair: Keypair) {
// First, get or create sender's token account
const senderATA = await getOrCreateAssociatedTokenAccount(
connection,
senderKeypair, // payer
TOKEN_MINT,
senderKeypair.publicKey
);
for (const recipient of recipients) {
try {
const recipientPubkey = new PublicKey(recipient.address);
// Get or create recipient's token account
const recipientATA = await getOrCreateAssociatedTokenAccount(
connection,
senderKeypair, // payer for account creation
TOKEN_MINT,
recipientPubkey
);
// Create transfer instruction
const transferIx = createTransferInstruction(
senderATA.address,
recipientATA.address,
senderKeypair.publicKey,
recipient.amount * 10 ** 9 // Assuming 9 decimals, adjust if different
);
// Send and confirm transaction
const tx = new Transaction().add(transferIx);
const signature = await sendAndConfirmTransaction(connection, tx, [
senderKeypair,
]);
console.log(`Sent ${recipient.amount} tokens to ${recipient.address}`);
console.log(`Token Account: ${recipientATA.address.toString()}`);
console.log(`Transaction: https://explorer.solana.com/tx/${signature}`);
} catch (err) {
console.error(`Failed to send to ${recipient.address}:`, err);
}
}
}
// Usage example
const connection = new Connection("<YOUR_HELIUS_ENDPOINT>");
// NEVER HARDCODE PRIVATE KEYS IN PRODUCTION!
// This is just for demonstration purposes
const secretKey = bs58.decode("<YOUR_BASE58_ENCODED_PRIVATE_KEY>");
const senderKeypair = Keypair.fromSecretKey(secretKey);
airdropTokens(connection, senderKeypair)
.then(() => console.log("Airdrop complete!"))
.catch(console.error);
Airdrop Costs
A major challenge with airdrops on Solana is that each user address needs its own specialized token account. An Associated Token Account (ATA) links a user’s wallet address to a specific token mint. If someone doesn’t have an ATA for a given token, you have to create one for them. That creation costs around 0.002 SOL because you’re paying rent to store data on the blockchain (i.e., address X holds this amount of token Y). This might not be a big deal if you only have a few hundred recipients. But if you have tens of thousands, those fees add up fast.
Traditional Airdrop Costs
- Base Transaction Fee: 0.000005 SOL per address.
- Token Account Creation: ~0.002 SOL for each new account.
Let’s do some quick math for 10,000 recipients:
- With New Accounts: You’d spend about 20 SOL total.
At 1 SOL = 240 USD, that translates to 4,800 USD. - Without New Accounts: You’d pay roughly 0.05 SOL (only for transfers).
At the same conversion rate, 0.05 SOL is 12 USD.
If SOL’s price rises or you plan to reach 100,000 or 1 million addresses, these costs become monumental—especially when we realize we haven’t even considered priority fees yet.
Solution: ZK-Compressed Airdrops
With ZK Compression, you don’t create a full on-chain account for each user. Instead, you bundle everyone’s data into a single hash (i.e., a Merkle root) backed by zero-knowledge proofs. This approach:
- Skips paying rent for individual token accounts.
- Replaces thousands of on-chain accounts with one on-chain root.
For those same 10,000 recipients, costs might drop to about 0.01 SOL total–at the current rate, 0.01 SOL is just 2.40 USD. When you scale beyond that, the savings grow even more, and you still keep Solana’s security.
Tip: Check out the AirShip calculator to see how much ZK compression can cut your airdrop costs.
How Does ZK Compression Reduce Airdrop Costs?
Essentially, ZK Compression stores token data off-chain while keeping a small "fingerprint" (a hash) on-chain. This fingerprint references the ledger data, which leverages Solana’s security. However, it avoids creating a standard on-chain account for every wallet, cutting fees by more than 95%.
For the same 10,000 recipients, a ZK-compressed airdrop would cost ~0.01 SOL.
That's a massive reduction in costs from ~20 SOL.
The savings are even more significant once you scale to millions of addresses.
How Does ZK Compression Work?
Let’s look at a few terms to understand how ZK Compression works.
1. Merkle Trees
A Merkle tree is a data structure that organizes many pieces of data—like thousands of user balances—into a single, compact hash called the root.
Each “leaf” in a Merkle tree represents a piece of data (e.g., a compressed account). You combine (i.e., hash) pairs of leaves to get a “parent” hash and then combine parent hashes until you end up with one final hash — the root. This root is all you need to verify that any individual data (i.e., an account balance) stored in the leaves is authentic and unchanged.
Why Does It Matter for ZK Compression?
Instead of storing every user balance or token account in individual on-chain accounts, you store only the Merkle root on-chain. The underlying data, such as each user’s token balance, is kept within Solana’s ledger. This ledger data derives the current state and remains verifiable through the Merkle root.
2. Off-chain Storage
“Off-chain” in this context means the complete data (e.g., each user’s detailed account info) isn’t stored in traditional on-chain accounts. Instead, the data is recorded in Solana’s ledger in a more cost-efficient form. Specialized indexers track and manage this data, ensuring it can be efficiently accessed and verified against the on-chain Merkle root.
How Can We Trust “Off-chain/Ledger” Data?
Because the Merkle root is stored on-chain, anyone can verify the off-chain data by checking it against that root. If someone tries to change a user’s balance off-chain, the updated balance won’t match the on-chain Merkle root. The proof would fail, and the runtime would reject the transaction.
This lets you store large amounts of data (like millions of accounts) without paying rent for each one, yet remain certain it’s all correct.
3. Zero-Knowledge Proofs (ZKPs)
Zero-knowledge proofs (ZKPs) let you prove a statement without revealing all its details. Think of it like showing a friend you know what’s inside a sealed box without opening it. They can verify that your claim is valid without either of you ever opening up the box. On Solana, this means that you can prove an account’s balance aligns with the Merkle root without uploading any of the data on-chain.
Why Use ZK Proofs?
There’s a few reasons why:
- Small Proof Size: ZK proofs on Solana are becoming increasingly efficient, with many now compact enough to fit within Solana's transaction size limits (around a few hundred bytes). This efficiency allows for scalable, low-cost verification without overloading the network.
- Reduced On-chain Load: Instead of storing every account update on-chain, you submit a small proof referencing the Merkle root. When an update occurs, the root changes to reflect the new state, but you still avoid storing all the detailed data directly on-chain.
- Security: The proof confirms your off-chain data matches what’s “fingerprinted” by the root on-chain. If it doesn’t match, the proof fails, and the transaction is rejected.
ZK proofs tie everything together. You only keep the root on-chain and rely on proofs to show that any changes to the off-chain data are legitimate.
How Does ZK Compression Save Money?
There are three main ways ZK Compression saves Solana developers money:
1. No Separate Token Account for Every Wallet Address
Each user typically needs an on-chain token account in a standard Solana airdrop. That account costs rent. With ZK compression, you don’t create thousands of separate accounts. Instead, you batch them into a single Merkle tree.
2. Lower Rent
The blockchain only stores one Merkle root, not the complete data for each user. So your state storage (i.e., the “rent”) is drastically cheaper.
ZK Compression Airdrop Example
Imagine you have 10,000 users you want to airdrop tokens to. Here’s how the costs would breakdown for the traditional airdrop approach compared to using ZK Compression
Traditional Approach:
- You create up to 10,000 on-chain token accounts, paying rent for each.
- Each account creation requires a small but noticeable fee.
ZK Compression Approach:
- You build a Merkle tree off-chain that covers all 10,000 balances.
- You submit a single “root” of that tree on-chain.
- Now, you only maintain and update that one root with each airdrop action, proven by ZK proofs.
Even if you need multiple Merkle trees to handle a very large user base, you’re still storing far less data on-chain than you would with separate token accounts for everyone. That’s where the significant savings come from.
In short:
- Merkle Trees compress large sets of accounts into a single hash (the root).
- Off-chain storage cuts on-chain rent costs.
- ZK Proofs guarantee the off-chain data is valid without putting all details on-chain.
This combination frees you from paying rent for each user’s account, making large-scale airdrops or massive user bases much more affordable on Solana.
How to Airdrop Tokens with ZK Compression Using AirShip
AirShip is a free, open-source tool built for ZK-compressed airdrops. It has two main options:
- Web UI: The easiest way to send. It runs in your browser—no setup is needed.
- CLI: It’s faster, more secure, and runs locally instead of a browser.
Both handle the ZK Compression details for you. They work with RPC endpoints that support compressed tokens. You can get yourself a free endpoint at helius.dev.
Prerequisites
- A Solana wallet that holds the tokens you want to distribute.
- Enough SOL to cover the minimal fees.
- A list of recipient addresses (e.g. CSV file, NFT holders, or token holders). We recommend using DAS (Digital Asset Standard) to fetch asset holder data efficiently. For more details, visit the Helius DAS API Documentation.
- An RPC that supports ZK compression and the DAS API.
Using the Web UI
1. Go to the AirShip site and click “Create New Airdrop.”
Visit the AirShip website and click the “Create New Airdrop” button.
Using the Airship tool, you can also calculate the expected costs of your Airdrop and decompress compressed tokens in your wallet.
2. Enter your private key and RPC URL
Use an RPC like https://mainnet.helius-rpc.com/?api-key=YOUR_API_KEY.
Warning: Use a temporary wallet explicitly created for this airdrop task.
The private key is only used to streamline signing and sending transactions. It isn’t stored or shared anywhere, ensuring the security of your other assets.
3. Choose your recipients
Select NFT, token, or Saga holders, or upload your own CSV file.
4. Set the token amount
Specify the number or percentage of tokens you want to airdrop.
5. Review and confirm
Check the summary carefully, then confirm the airdrop.
AirShip will handle the batch sending and ZK proofs behind the scenes. The UI lets you see the transactions' processes and when they are completed.
Using the CLI
The CLI is better for larger airdrops or if you like working in a terminal.
1. Install via pnpm
npm install -g helius-airship
Or build from source:
git clone https://github.com/helius-labs/airship.git
cd airship
pnpm install && pnpm build
cd packages/cli
pnpm link --global
2. Run the CLI:
helius-airship \
--keypair /path/to/your_wallet.json \
--url "https://mainnet.helius-rpc.com/?api-key=YOUR_API_KEY"
3. Add your list of targets
If you follow the steps, AirShip will prompt you for your target recipients (CSV, etc.) and the token amount.
That’s it!
AirShip will now send batches of transactions to your airdrop recipients.
Conclusion
You can save a lot of money by using ZK Compression on Solana. Traditional airdrops often cost 20 SOL or more for 10,000 recipients (due to token account creation). With ZK compression, it can go down to 0.01 SOL. Tools like AirShip make it simple to launch these airdrops without writing your code from scratch.
Next Steps
- Check out AirShip on GitHub and give it a star!
- Read more about ZK compression on Light Protocol’s site.
- For more technical details, check out the article “ZK Compression Keynote: Breakpoint 2024”, which provides a thorough walk-through of how these proofs work, the Merkle structures, and how entire systems handle the updates.
ZK compression lets you reward your community or grow your user base without burning through your treasury. It keeps the cost low while keeping the security and speed of Solana. It’s a big step forward for large-scale token distribution.
Related Articles
Subscribe to Helius
Stay up-to-date with the latest in Solana development and receive updates when we post