Uploading Files to Shadow Drive on Solana
Overview
Solana's high cost and limited storage capacity make storing large amounts of data cost-prohibitive. Off-chain storage solutions like Shadow Drive, created by GenesysGo, have gained traction to overcome these limitations.
Shadow Drive provides a cost-efficient and performant option for storing large amounts of data on Solana. However, it requires a mainnet token, which you acquire by doing a SOL → SHDW swap.
In this tutorial, we will walk through uploading files to Shadow Drive using TypeScript.
Note: This test is only possible to run on mainnet, as SHDW does not have a devnet option currently available.
Prerequisites
Before getting started, make sure you have the following prerequisites installed:
Setting up the Environment
Creating Project
- Create a new folder from your terminal called Shadow.
- Create a new file called upload.ts inside the Shadow folder.
- Install the required packages:
4. Generate a wallet using the following command:
5. Create a file named "helius.txt" inside the "Shadow" folder and add some content to it. In this example, we are just placing the following:

After completing these steps, your file directory structure should resemble the following:
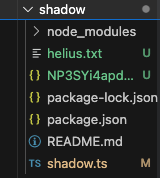
- Open the file named [walletAddress].json and copy the secret key. We will need to fund this wallet for the example.
- Install the Backpack Wallet extension for your browser from the Chrome Web Store.
- Open the Backpack Wallet extension and go to "Settings".

4. In the settings menu, select "Wallets".
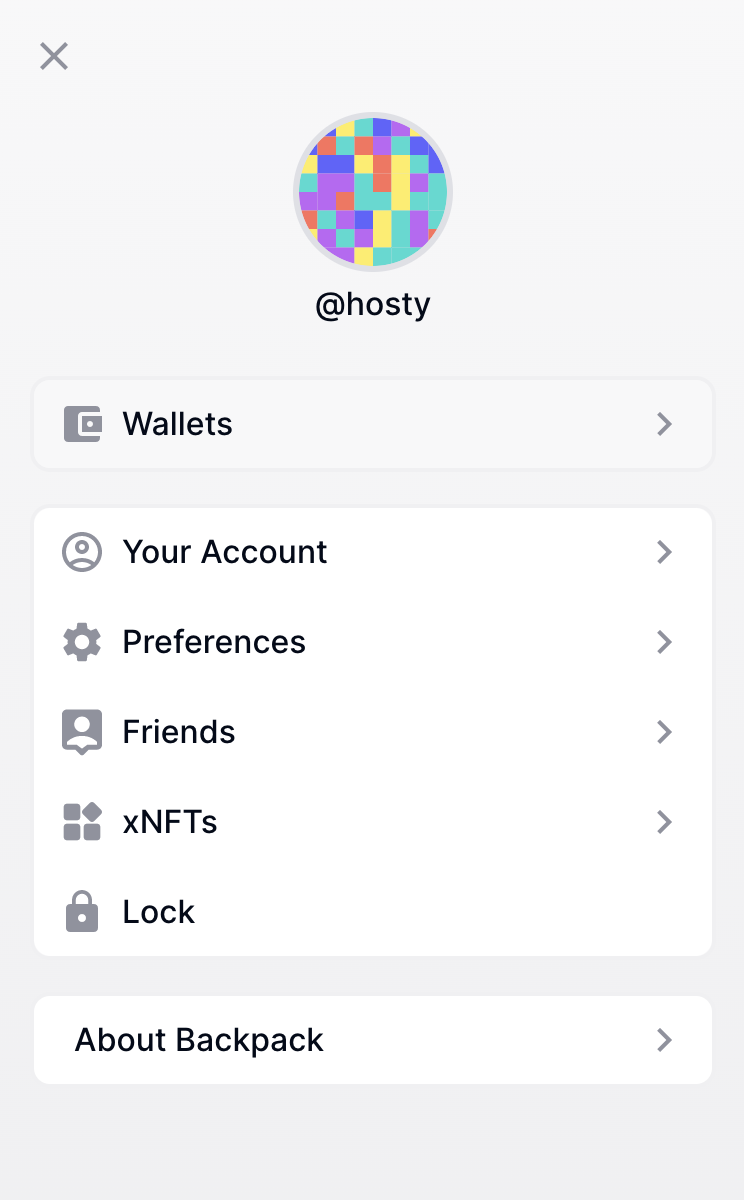
5. Click on the "+" button at the top right to add a new wallet.
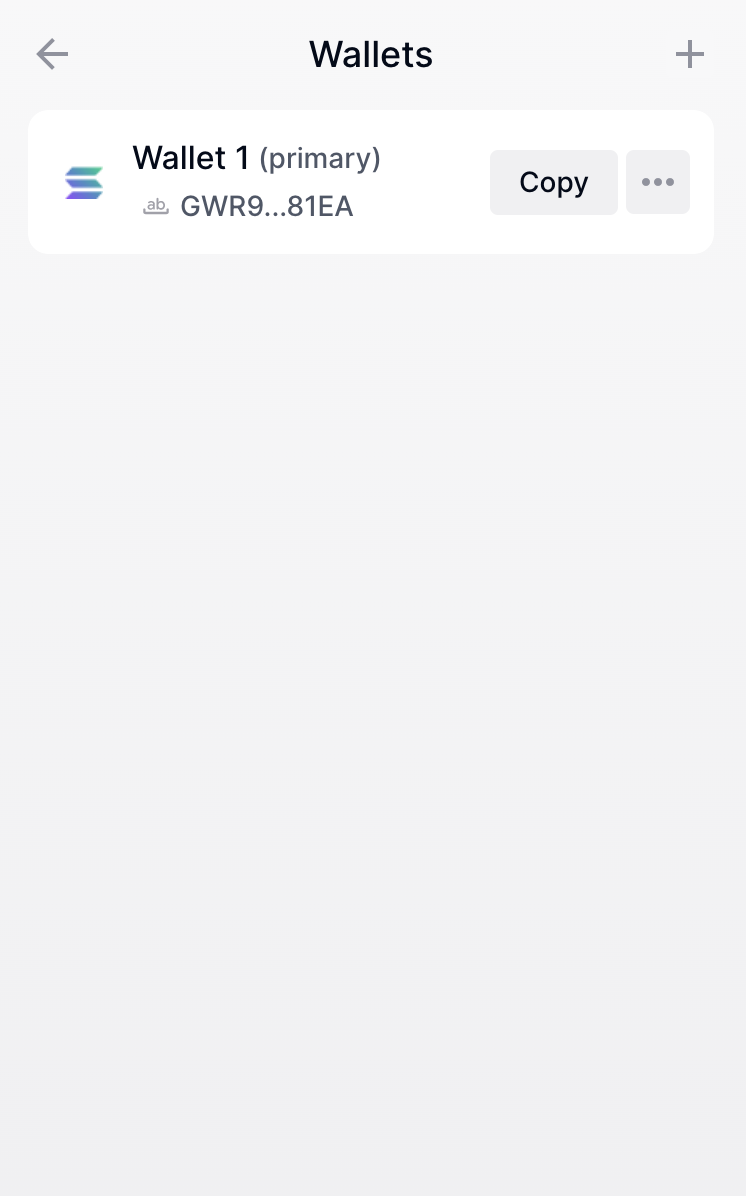
6. Choose Solana as the wallet type and select "Import by Private key".
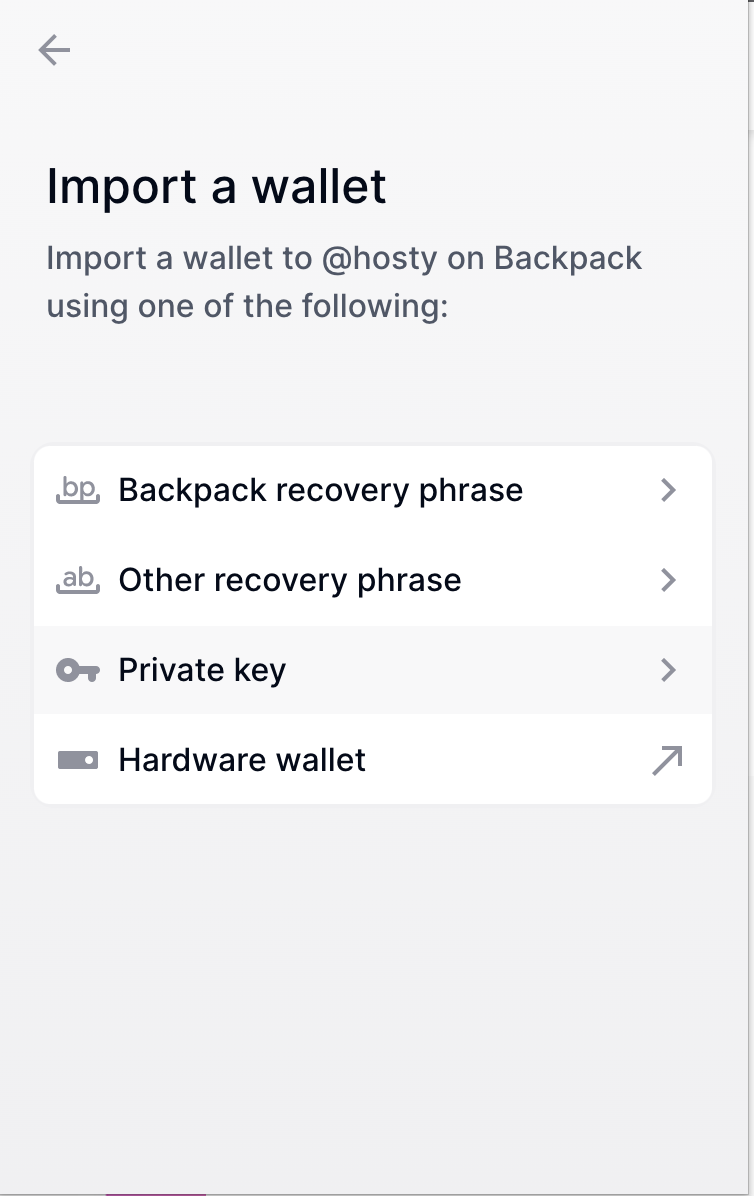
7. Paste the contents of the wallet file into the import field and click "Import".

Once imported, copy the public key from the wallet. You can fund this test wallet with less than 0.03 SOL from another Solana wallet.
Getting SHDW Token
SHDW Drive requires a token called SHDW (SHDWyBxihqiCj6YekG2GUr7wqKLeLAMK1gHZck9pL6y) to create storage accounts. You can obtain SHDW tokens through a swap process using Jupiter on Solana.
- Visit https://jup.ag/swap/SOL-SHDW to perform the SOL to SHDW token swap.
- Enter the desired amount of SOL to swap for SHDW, and click "Swap". We only need less than 1 SHDW for this test (which equates to .01 SOL).
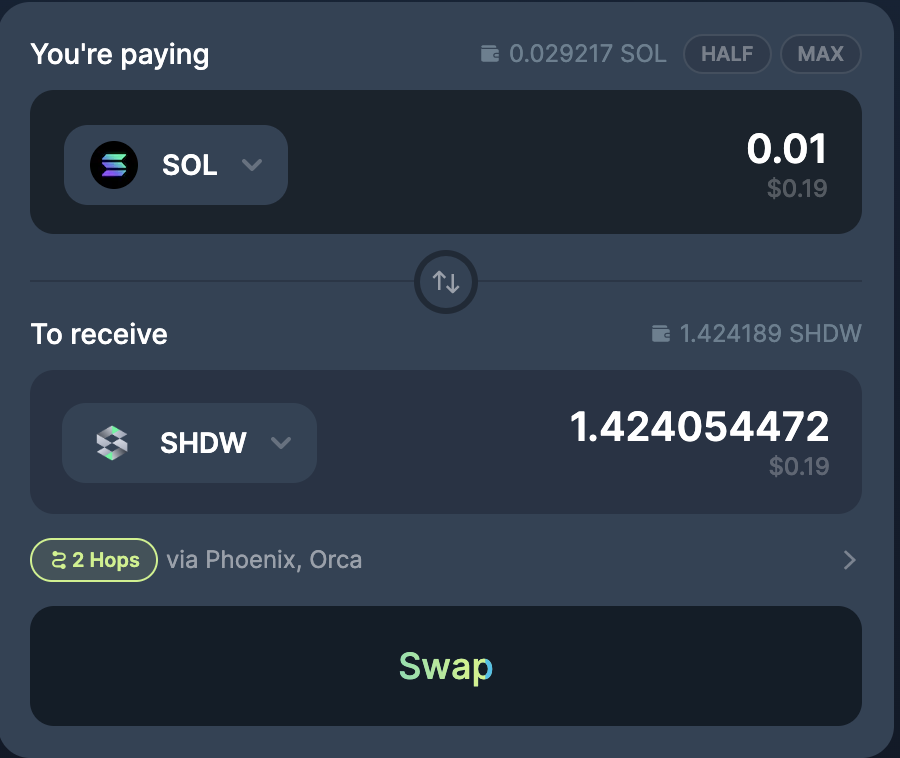
3. Confirm the transaction. After the transaction is approved, the SHDW token balance will reflect in your wallet.
Steps to Build
1. Setting Connection to SHDW
In your upload.ts file, we'll import the necessary modules and establish a connection to the SHDW network. We'll create an asynchronous function named main to nest our code:
Ensure to replace api-key in the rpc variable with a valid API key that you can obtain from the Helius Developer Portal. Also, substitute const key with the correct filename of your wallet we created before starting.
If you were to run the file now with ts-node upload.ts you will see the following produced in your terminal:
2. Create a Storage Account
To create a storage account, add the following code inside the main function:
Running the ts-node upload.ts command here will output the newly created storage account's ID and transaction signature.
Note: You may encounter an error at this step if the wallet is not funded with at least .01 SOL and 1 SHDW token.
3. Upload a File
Once we have a storage account, we can upload a file to it. Add the following code inside the main function:
The code above performs four primary actions:
- It reads the local file helius.txt as a buffer.
- Finds the storage account we created, and defines it as our account public key.
- It creates a ShadowFile object with the file's name and data.
- It uploads the file to the specified storage account using the uploadFile function.
We can now run the following to begin our upload:
Which produces the following in our console:
Now the file is uploaded! You can visit the finalized_location link produced, and make sure the file was uploaded correctly.
Notice the url for the file is https://shdw-drive.genesysgo.net/[storage_account_address]/[file_name]
Meaning any file uploaded to this storage account will share the initial URL, but the file name will change. You can also create separate storage accounts to store files.
You will also notice after checking your wallet: this operation only cost .00244 SHDW to complete.
Full Code
Conclusion
That's it! You've successfully uploaded a file to Shadow Drive using TypeScript. This fundamental knowledge enables you to take advantage of SHDW's decentralized capabilities and provides a foundation for more advanced use cases.For instance, you could extend this tutorial by integrating file upload functionality into a web application or creating a service for decentralized file storage.Please check out the official docs for a further look into what you can do with the Shadow Drive SDK.