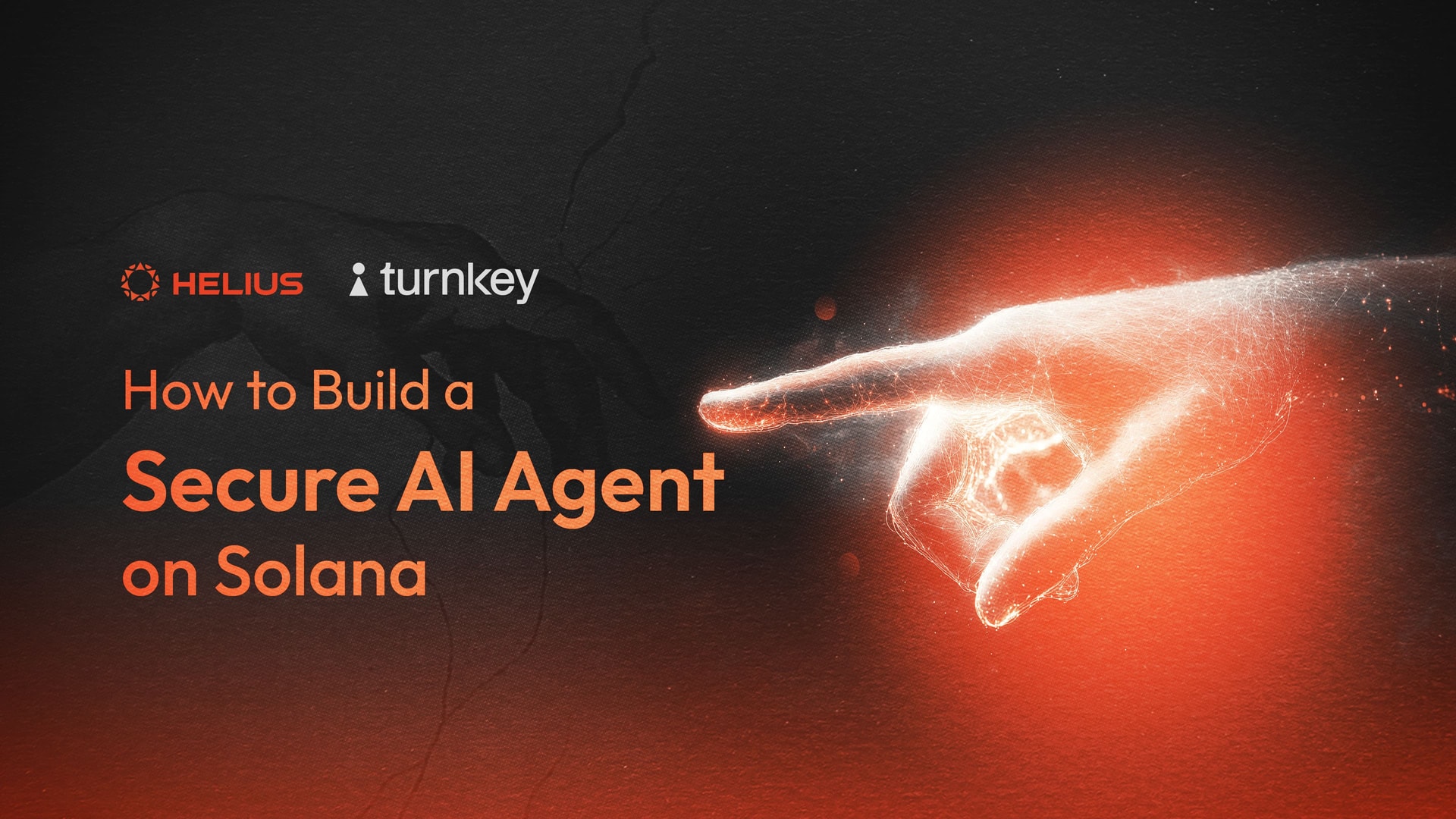
How to Build a Secure AI Agent on Solana
The surge of AI agents trading on Solana has sparked intense speculation about autonomous agents conducting financial transactions.
The current wave of AI tokens is generating $1B+ in 24-hour trading volume and represents over $9.5B in market capitalization.[1] But beyond these speculative markets, a deeper shift is already underway in crypto — one built on years of increasing automation.
Bots already drive a large majority of crypto transactions, as much as 90% in some segments of crypto.[2] These aren’t fully autonomous AI agents — yet. But they are clear precursors, growing more general and independent over time.
Their prevalence underscores something crucial: crypto infrastructure uniquely suits programmatic, autonomous transactions.
The Security Challenge: How can AI Agents access Solana wallets safely?
With this rise of AI agents on Solana and beyond, a major security challenge has emerged: how can autonomous systems access wallets without compromising security?
To execute onchain actions, an AI agent needs wallet access. But private keys stored in code create massive vulnerabilities:
- They’re prime targets for attackers
- They’re susceptible to code errors that could lead to lost funds
- Developers rarely structure them in a way that segregates funds
- They could open the door to rogue AI behavior
A real-world example of these risks occurred in September 2024, when attackers exploited a vulnerability in Banana Gun, a Telegram-based trading bot. A flaw in its message oracle allowed hackers to drain $3M from users' wallets.
A bot or agent with direct wallet access can make unintended, irreversible transactions whether due to an exploit, a bug, or an unchecked decision loop.
Balancing Security and Autonomy
Traditional solutions force developers to choose between security and true agent autonomy. Traditional approaches either expose private keys or require centralized custody, neither of which is acceptable for production systems.
One solution is offered by Turnkey, a highly flexible key management infrastructure that is purpose-built for security, scale, and automation. Instead of exposing private keys, Turnkey enables AI agents to interact with wallets through scoped, policy-controlled API access.
Here’s how it works:
Scoped API keys
AI agents receive limited API credentials that are tied to specific wallets and actions.
Fine-grained policies
Developers can define exactly what an AI agent is allowed to do — whether that’s signing transactions under certain conditions, interacting with specific smart contracts, or enforcing rate limits.
User control
End users or developers can retain full authority over wallets while delegating specific actions to AI agents.
Why this Works:
This approach ensures AI agents can autonomously interact with on-chain assets without ever holding raw private keys, solving the security problem while preserving crypto's trustless, permissionless nature.
Additionally, Turnkey's verifiable computing environment allows teams to deploy AI agents in an environment where anyone can verify the code being run. More on this later.
Let’s walk through a practical example of provisioning an API key with wallet permissions to a simple bot. From there, the developer can take the next step: evolving the bot into a fully autonomous AI agent.
How to Create a Solana AI Agent
Let’s build a secure trading bot using Turnkey. We’ll cover:
- Setting up a Solana wallet with policy controls
- Creating an API-only user for the bot
- Defining policies to constrain the bot’s behavior
- Implementing a simple trading function using Jupiter Exchange
Prerequisites
Before diving into the code, you must have Node.js and npm installed.
You’ll also need a Turnkey account.
Create a Solana Wallet in Turnkey
Log into your Turnkey dashboard and then:
- Navigate to Wallets
- Click Create New Wallet
- Select Solana and ED25519 as your wallet settings
- Fund this wallet with some SOL for trading
Create an API-only User for Your Bot
Next:
- Go to the Users tab
- Click Add User
- Select API key under access types
- Give it a name (e.g. "Trading Bot Alpha")
Write down the user ID and then click approve. You'll need this key for your policy configuration.
Limit Your Bot via Policies
First, navigate to the Policies tab and click Add new policy. Then, configure a policy to limit the actions the bot can take. Let’s define a policy that ensures the bot:
- Can only execute token transfers (no other transaction types)
- Can only trade SOL and USDC
- Cannot execute transactions larger than 1 SOL
{
"policyName": "AI Trading Bot Policy",
"effect": "EFFECT_ALLOW",
"consensus": "approvers.any(user, user.id == '<BOT_USER_ID>')",
"condition": "solana.tx.instructions.count() == solana.tx.spl_transfers.count() && solana.tx.spl_transfers.all(transfer, transfer.token_mint == '<USDC_MINT>' || transfer.token_mint == '<SOL_MINT>') && solana.tx.spl_transfers.all(transfer, transfer.amount < 1000000000)"
}
Set up Your Project
Next, create your project and install dependencies.
mkdir ai-trading-bot
cd ai-trading-bot
npm init -y
npm install @solana/web3.js @turnkey/sdk-server @turnkey/solana @jup-ag/api
Create a .env file to store your credentials.
TURNKEY_API_PRIVATE_KEY="YOUR_API_PRIVATE_KEY_HERE"
TURNKEY_API_PUBLIC_KEY="YOUR_API_PUBLIC_KEY_HERE"
TURNKEY_ORGANIZATION_ID="YOUR_TURNKEY_ORGANIZATION_ID_HERE"
Create a Simple Bot
Now, let’s write the code. Create an index.js file:
import process from "node:process";
import { Turnkey } from "@turnkey/sdk-server";
import { TurnkeySigner } from "@turnkey/solana";
import {
Connection,
clusterApiUrl,
PublicKey,
VersionedTransaction
} from "@solana/web3.js";
import { createJupiterApiClient } from "@jup-ag/api";
// Load environment variables
process.loadEnvFile(".env");
// Define token addresses
const USDC = "EPjFWdd5AufqSSqeM2qN1xzybapC8G4wEGGkZwyTDt1v";
const SOL = "So11111111111111111111111111111111111111112";
const BOT_ADDRESS = "YOUR_TURNKEY_WALLET_ADDRESS_HERE";
const BOT_PUBLIC_KEY = new PublicKey(BOT_ADDRESS);
async function main() {
// Initialize Turnkey and Jupiter clients
const turnkey = new Turnkey({
apiBaseUrl: "https://api.turnkey.com",
apiPrivateKey: process.env.TURNKEY_API_PRIVATE_KEY,
apiPublicKey: process.env.TURNKEY_API_PUBLIC_KEY,
defaultOrganizationId: process.env.TURNKEY_ORGANIZATION_ID,
});
const signer = new TurnkeySigner({
organizationId: process.env.TURNKEY_ORGANIZATION_ID,
client: turnkey.apiClient(),
});
const connection = new Connection(clusterApiUrl("mainnet-beta"), "confirmed");
const jupiterClient = createJupiterApiClient();
Create a Policy-controlled Credential
Now, here’s where it gets interesting. Instead of giving your AI agent full wallet access, we’ll create a policy-controlled credential:
const agentUSDCPolicy = {
"policyName": "Require consensus on Solana transactions containing SPL token transfers of over 1000 USDC",
"effect": "EFFECT_ALLOW",
"consensus": "approvers.count() >= 2",
"condition": "solana.tx.spl_transfers.all(transfer, transfer.token_mint == 'EPjFWdd5AufqSSqeM2qN1xzybapC8G4wEGGkZwyTDt1v') && solana.tx.spl_transfers.all(transfer, transfer.amount > 1000000000)",
"notes": "",
}
const agentSOLPolicy = {
"policyName": "Require consensus on Solana transactions containing SOL transfers over 1 SOL",
"effect": "EFFECT_ALLOW",
"consensus": "approvers.count() >= 2",
"condition": "solana.tx.transfers.count == 1 && solana.tx.transfers[0].amount > 1000000000",
"notes": "",
}
const createUSDCPolicyResponse = await turnkey.apiClient().createPolicy(agentUSDCPolicy);
const createSOLPolicyResponse = await turnkey.apiClient().createPolicy(agentSOLPolicy);
Implement the Bot’s Trading Function
With the constraints in place, you can implement your trading function:
async function executeTrade(fromToken: string, toToken: string, amount: string) {
// Get quote from Jupiter
const quoteResponse = await jupiterClient.quoteGet({
inputMint: fromToken,
outputMint: toToken,
amount: amount
});
// Create swap transaction
const swapResponse = await jupiterClient.swapPost({
swapRequest: {
userPublicKey: BOT_PUBLIC_KEY,
quoteResponse: quoteResponse,
},
});
// Sign and submit transaction
const transaction = VersionedTransaction.deserialize(
Buffer.from(swapResponse.swapTransaction, "base64")
);
const signedTx = await signer.signTransaction(
transaction,
BOT_PUBLIC_KEY
);
const txid = await connection.sendRawTransaction(
signedTx.serialize(),
{ skipPreflight: true, maxRetries: 5 }
);
await connection.confirmTransaction(txid);
return txid;
}
main();
And there you have it!
It’s not a full-fledged AI agent yet — but this simple, policy-controlled bot lays the groundwork.
From here, you can extend its autonomy, add decision-making logic, and evolve it into a more sophisticated agent.
You can also continue to enhance security by requiring multi-sig approvals for high-stake transactions or granting agents access to wallets controlled by end-users.
Beyond Secure Wallets: How Verifiable Environments Unlock Secure AI Agents
Ensuring AI agents transact securely isn’t just about protecting wallet access—it’s about verifying that an agent is running the code it claims to be running.
Imagine a world where AI agents transact securely and operate in a fully verifiable environment. In this environment, anyone can independently confirm exactly what code an agent runs.
Turnkey’s verifiable computing infrastructure enables anyone to verify what software is running inside a secure enclave — solving one of the biggest challenges in trusted software today.
At its core, Turnkey leverages Trusted Execution Environments (TEEs), specifically AWS Nitro Enclaves, to provide a tamper-proof environment for running sensitive operations. These enclaves operate as isolated virtual machines with no persistent storage, no external network access, and no ability to be modified after launch. This guarantees that once an enclave is provisioned, its code and data remain secure from external interference—including from the infrastructure provider itself.
However, secure execution alone isn’t enough—what matters is verifiability. Turnkey achieves this through three key innovations:
1. Remote Attestations
Every Turnkey enclave generates a cryptographic proof that certifies precisely what code it is running. This attestation includes a hash of the enclave’s operating system and application binary, signed by AWS’s Nitro Secure Module (NSM). Soon, anyone can independently verify this proof to confirm that the enclave runs the expected software.
2. QuorumOS (QOS)
A minimal, open-source operating system designed specifically for verifiability. QOS ensures that every enclave runs only approved, auditable code and provides QOS Manifests—machine-readable proofs that link an enclave’s execution to a publicly verifiable software fingerprint.
3. StageX & Reproducible Builds
To eliminate the risk of supply chain attacks, Turnkey enforces reproducible builds. Any binary running inside an enclave can be independently recompiled from human-readable source code to verify that it hasn’t been altered. This ensures a 1:1 mapping between code reviewed by developers and the software running inside a secure environment.
By combining TEEs, remote attestations, and reproducible builds, Turnkey makes it possible to run applications with absolute verifiability—whether for wallet security, cryptographic signing, or AI agent execution. This architecture moves beyond traditional cloud security models, where trust is assumed, to a new paradigm where security is provable, transparent, and decentralized.
Why This Matters for AI Agents
A verifiable execution environment improves security and broadens the design space for autonomous systems, financial applications, and any service requiring provable trust.
When users can independently verify the software running in an enclave, new possibilities emerge — like trustless AI execution where developers and users can confidently avoid “Wizard of OZ” agents (i.e. human actors pretending to be real AI agents).
Beyond verifiable AI, teams can deploy everything from offchain co-processors to trusted oracles and AI inference engines. All with cryptographic proof that they’re executing exactly as promised and cannot be unilaterally updated. Some examples of sensitive workloads that could benefit from moving to attestable environments are in the table below:
Want to get involved and run your own critical applications verifiably?
The Turnkey team is now partnering with select teams to deploy AI agents and other applications in a fully verifiable execution environment—where anyone can validate the exact code running behind the scenes.
Additional Resources
- CoinGecko — AI Agent Market Capitalization
- QZ — Bots make 90% of stablecoin transactions
- Turnkey's new whitepaper
- Turnkey Official Site
- Get in touch to explore deploying verifiable AI
Related Articles
Subscribe to Helius
Stay up-to-date with the latest in Solana development and receive updates when we post