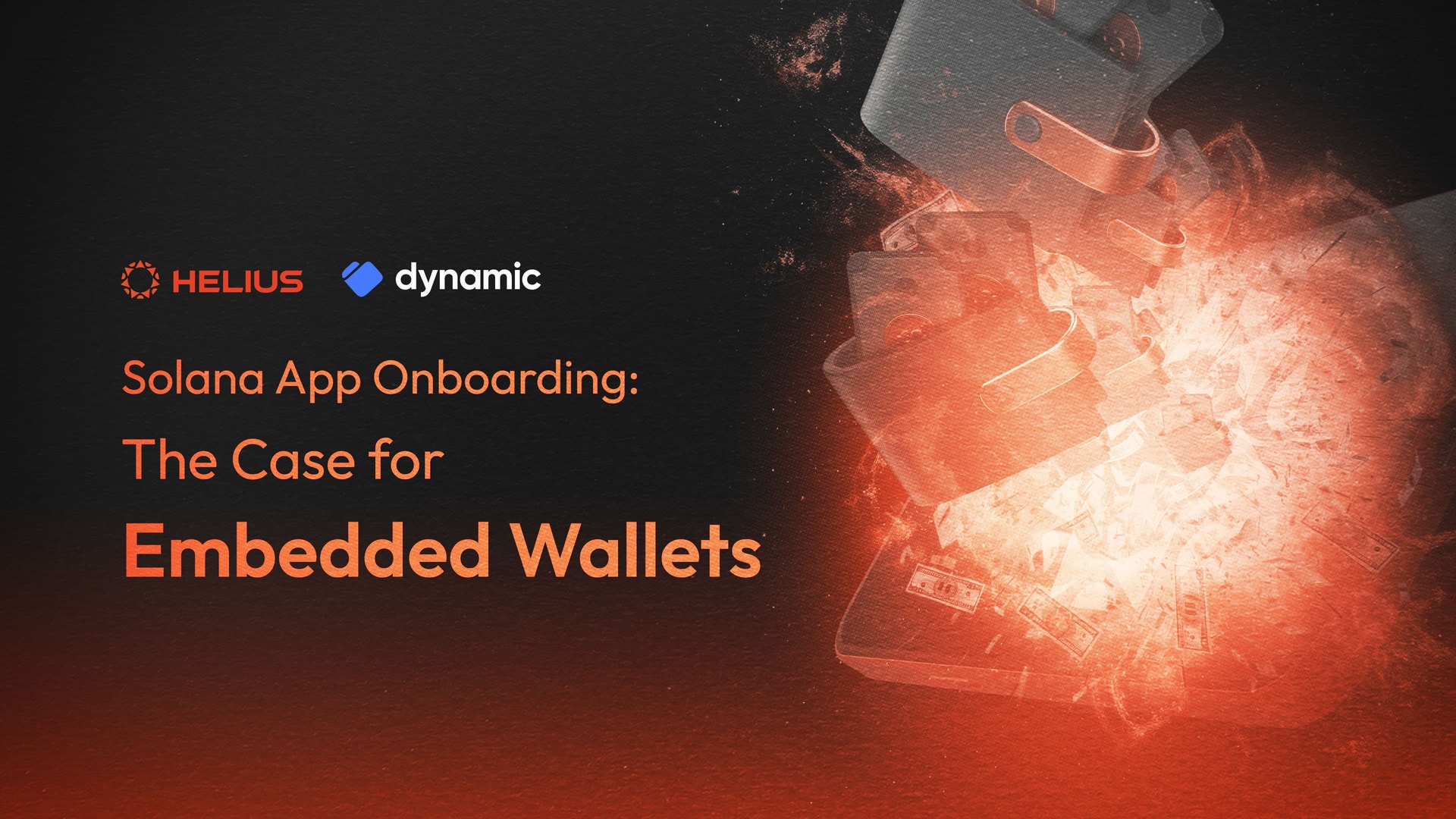
Solana App Onboarding: The Case for Embedded Wallets
You’ve got a groundbreaking idea for a mobile or web app.
You know Solana is the perfect blockchain to build on, and you’re eager to launch quickly with a feature-packed experience that will captivate users from day one.
So, what’s in your developer toolkit to make it happen?
Tools like Cursor and v0 are likely high on your list, and Next.js is a strong contender for your frontend framework of choice.
But when it comes to meeting the Solana-specific demands of your app, what solutions will help you ensure a smooth launch and the ability to scale effectively?
Building and scaling on Solana requires two critical components:
- Seamless onboarding solution for auth and wallet management
- Robust RPC services to handle high-performance transactions
These essentials not only simplify development but also provide a seamless user experience.
Let’s dive deeper into each of these elements.
Solana Wallet Adapter
The simplest onboarding process is to connect users through an existing wallet such as Phantom or Solflare. This method works well for onboarding users who are familiar with Solana and have wallets set up.
A good starting point is the Solana Wallet Adapter, which offers:
- A standardized API for straightforward integration
- Basic functionality for wallet connections and transaction signing
- Open-source flexibility, to customize it to your app’s needs
The Solana Wallet Adapter is fast and simple to implement, making it a reliable choice for users with existing wallets.
In addition to supporting multiple wallets, it is also equipped with standardized integration for consistent wallet functionality and ready-to-use UI components such as wallet connection buttons. Lastly, it optimizes performance for faster transaction signing and sending compared to raw APIs like window.solana
.
The open-source design of the Solana Wallet Adapter allows developers to customize it to specific app requirements. It also enables community implementations to extend its compatibility to frameworks such as Vue, Angular, Svelte, and TypeScript.
However, using the adapter does introduce additional dependencies to your project, which can add overhead for developers.
While the adapter provides many features out of the box, achieving extensive customization may require diving into its codebase or creating workarounds. Depending on the use case, this could be time-consuming.
As with any third-party library, developers may encounter bugs or issues outside their control, potentially impacting app stability or requiring external support to resolve.
Although using a wallet adapter is practical for crypto-native users, it falls short when your goal is to onboard a broader audience, especially users unfamiliar with blockchain or those who don’t yet have a wallet.
Onboarding New Users with Embedded Wallets
Embedded wallets can significantly enhance Solana onboarding, enabling developers to acquire more users. Embedded wallets allow new users to sign up, and automatically receive a wallet within the app itself.
This allows users to:
- Get started without setting up a third-party wallet
- Seamlessly receive assets
- Make on-chain transactions
This approach minimizes friction and is valuable for consumer apps targeting mainstream adoption.
According to data from Dynamic, more than 50% of users opt for an embedded wallet rather than connecting an existing one.
However, adoption rates vary depending on the onboarding method and target user base.
Crypto-native audiences may lean toward connecting their existing wallets, while more mainstream users will likely prefer the convenience of embedded solutions.
Finding the right balance is key.
Retail liquidity still resides primarily in hot wallets, meaning that while embedded wallets streamline onboarding, they may not always be the best starting point. Developers should consider their initial target audience and design an onboarding experience that aligns with their users’ needs.
A Comprehensive SDK for Onboarding and Wallet Infrastructure
For developers looking to enable features beyond what the Solana Wallet Adapter provides, solutions like Dynamic’s SDK offer a more comprehensive approach.
With Dynamic, you can:
1. Support multiple wallet options
Allow users to connect existing Solana wallets like Phantom and issue embedded wallets for new users.
2. Enable social and email logins
Simplify wallet setup with familiar login methods such as X and Telegram.
3. Customize user experiences
Use prebuilt UI components for rapid integration or opt for fully headless options to design your own flows.
4. Future-proof your app
Add multi-wallet support, fraud protection tools, and analytics capabilities for scaling and optimizing your application.
5. Capture users from other chains
Expand your app’s functionality beyond Solana to other chains when you’re ready.
Using a solution like Dynamic, you can streamline development while providing users with a seamless onboarding experience.
It complements the Solana Wallet Adapter by giving developers more onboarding functionality, scalability, and customization are key.
Solana Wallet Adapter vs. Embedded Wallets
Here’s a helpful comparison:
Feature | Solana Wallet Adapter | Dynamic |
Wallet connections | ✅ | ✅ |
Embedded wallets | ❌ | ✅ |
Social logins | ❌ | ✅ |
Multichain support | ❌ | ✅ |
Customizable UI | ❌ | ✅ |
Fraud protection | ❌ | ✅ |
Access lists | ❌ | ✅ |
User management | ❌ | ✅ |
Webhooks | ❌ | ✅ |
Regardless of features, the implementation time will always be a dealbreaker, and you might think that with this much going on, the implementation of Dynamic vs the Solana Wallet Adapter is more complicated, but this is not the case.
Below is a comparison of the default React implementations:
Solana Wallet Adapter: Default Implementation
import React, { FC, useMemo } from 'react';
import { ConnectionProvider, WalletProvider } from '@solana/wallet-adapter-react';
import { WalletAdapterNetwork } from '@solana/wallet-adapter-base';
import { UnsafeBurnerWalletAdapter } from '@solana/wallet-adapter-wallets';
import {
WalletModalProvider,
WalletDisconnectButton,
WalletMultiButton
} from '@solana/wallet-adapter-react-ui';
import { clusterApiUrl } from '@solana/web3.js';
// Default styles that can be overridden by your app
require('@solana/wallet-adapter-react-ui/styles.css');
export const Wallet: FC = () => {
// The network can be set to 'devnet', 'testnet', or 'mainnet-beta'.
const network = WalletAdapterNetwork.Devnet;
// You can also provide a custom RPC endpoint.
const endpoint = useMemo(() => clusterApiUrl(network), [network]);
const wallets = useMemo(
() => [
new UnsafeBurnerWalletAdapter(),
],
// eslint-disable-next-line react-hooks/exhaustive-deps
[network]
);
return (
<ConnectionProvider endpoint={endpoint}>
<WalletProvider wallets={wallets} autoConnect>
<WalletModalProvider>
<WalletMultiButton />
<WalletDisconnectButton />
{ /* Your app's components go here, nested within the context providers. */ }
</WalletModalProvider>
</WalletProvider>
</ConnectionProvider>
);
};
Dynamic: Default Implementation
import React from 'react'
import { DynamicContextProvider, DynamicWidget } from '@dynamic-labs/sdk-react-core'
import { SolanaWalletConnectors } from '@dynamic-labs/solana'
export const App = () => {
return (
<div className="App">
<DynamicContextProvider
settings={{
environmentId: process.env.REACT_APP_DYNAMIC_ENVIRONMENT_ID,
walletConnectors: [SolanaWalletConnectors],
}}
>
{ /* Your app's components go here, nested within the context providers. */ }
<DynamicWidget />
</DynamicContextProvider>
</div>
)
}
You're completely set up for onboarding since we’re using the DynamicWidget
, an all-in-one UI component that handles a Login/Signup button, full authentication flow, user profile, and more.
Upon starting the app, you’ll see the following:
If you prefer to build your own UI or customize specific elements, everything is fully configurable down to the pixel. Explore the design documentation or the headless integration option for detailed guidance.
To enable embedded wallet signups on Solana, simply navigate to the dashboard and follow these steps:
That’s it!
Anyone who signs up with an email, phone, or social media account will get a Solana wallet, and they can immediately use your app.
With a few lines of code, you get best-in-class onboarding, including all the functionality you need now and in the future.
Adding your RPC Provider and Setting Priority Fees
While onboarding remains one of the biggest friction points for users today, transaction processing comes in as a close second. Navigating network congestion on Solana can be tricky. Without a dedicated RPC service, users may experience dropped transactions, leading to frustration. Similarly, setting incorrect prioritization fees can disrupt the user experience and undermine the app's reliability.
You can avoid this simply by using Helius for both the RPC endpoint and setting fees dynamically using their Priority Fee API. Plugging it into Dynamic is as simple as setting the RPC URL in the dashboard.
When creating the transaction, fetch the fee using the Priority Fee API.
async function getPriorityFeeEstimate(priorityLevel, transaction) {
const response = await fetch(HeliusURL, {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({
jsonrpc: "2.0",
id: "1",
method: "getPriorityFeeEstimate",
params: [
{
transaction: bs58.encode(transaction.serialize()), // Pass serialized transaction in Base58
options: { priorityLevel: priorityLevel },
},
],
}),
});
const data = await response.json();
console.log(
"Fee in function for",
priorityLevel,
"",
data.result.priorityFeeEstimate
);
return data.result;
}
Magic Eden Case Study
A robust wallet infrastructure is crucial for developing powerful and scalable Solana apps.
Magic Eden, the leading NFT marketplace, has aggressively scaled by adding new chains. They needed a flexible wallet to meet this approach and excel during times of high traffic, such as NFT mints.
By integrating Dynamic’s SDK, Magic Eden can offer multi-chain wallet support, social linking, and multi-wallet management. Managing multiple wallets and collecting across networks is simple.
Magic Eden also relies on Helius to manage RPC nodes and optimize transaction throughput. Through Helius's Priority Fee API, Magic Eden maintains reliability and speed, even during peak traffic.
This combination of Dynamic and Helius has enabled Magic Eden to scale efficiently while providing a superior experience to its millions of monthly users.
By prioritizing scalability, speed, and user experience, Magic Eden sets a benchmark for what is possible for NFT marketplaces.
For developers looking to build apps that appeal to crypto-native and mainstream audiences, investing in a reliable wallet solution can streamline development, reach a broader user base, and lay the groundwork for long-term growth.
Additional Resources
Many thanks to @__lostin__ and Brady Werkheiser for reviewing earlier versions of this article.
Related Articles
Subscribe to Helius
Stay up-to-date with the latest in Solana development and receive updates when we post